Understanding Python Classes
Python classes provide all the standard features of Object Oriented Programming: the class inheritance mechanism allows multiple base classes, a derived class can override any methods of its base class or classes, and a method can call the method of a base class with the same name.
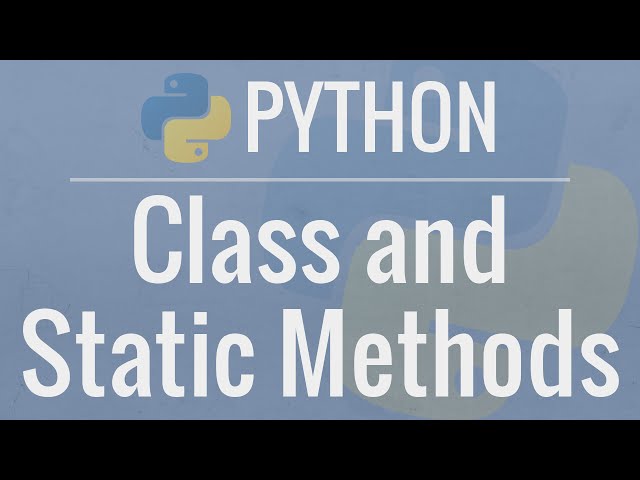
What is a Class?
In Python, a class is a blueprint for creating objects. It defines the attributes (data) and methods (functions) that the objects will have.
Creating a Class:
To create a class in Python, you use the class keyword followed by the class name. Here's a basic example:
class MyClass:
Pass
Constructor Method
The __init__() method is called a constructor and is used to initialize the object's state. It gets called automatically when you create a new instance of the class.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Creating Objects (Instances)
Once you've defined a class, you can create objects (instances) of that class using the class name followed by parentheses.
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
Instance Variables
Instance variables are unique to each instance of a class. You define them inside the __init__() method using self.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Instance Methods
Instance methods are functions that belong to objects created from the class. They can access and modify the instance variables.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f"Hello, my name is {self.name} and I am {self.age} years old."
Accessing Object Attributes and Calling Methods
You can access object attributes and call methods using the dot notation.
print(person1.name) Output: Alice
print(person2.age) Output: 25
print(person1.greet()) Output: Hello, my name is Alice and I am 30 years old.
Inheritance
Inheritance allows a class to inherit attributes and methods from another class. It promotes code reusability and enables the creation of a hierarchy of classes.
class Student(Person):
def __init__(self, name, age, student_id):
super().__init__(name, age)
self.student_id = student_id
Encapsulation
Encapsulation means hiding the internal state of an object and restricting access to it. In Python, you can achieve encapsulation by using private variables and methods.
class Car:
def __init__(self, speed):
self.__speed = speed
def get_speed(self):
return self.__speed
def set_speed(self, speed):
if speed > 0:
self.__speed = speed
Conclusion
Classes are a fundamental concept in object-oriented programming (OOP) and Python. They allow you to structure your code in a more organized and reusable way. As you become more familiar with Python, exploring classes and their various features will be essential for building complex applications.
What's Your Reaction?
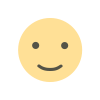
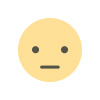
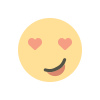
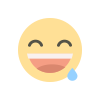
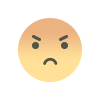
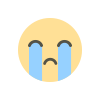
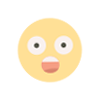